Execution/Test Class
Let us create an array with capacity
as 4
and insert 9
numbers in it, the size
of the array becomes 16
.
Code
Following is the execution class
Illustrations & Explanation
We gave our array-like data structure with 9
elements {1, 2, 3, 4, 5, 6, 7, 11, 9}
, But we initialized the size to 4
using Array array = new Array(4);
.
A simple illustration is as follows:
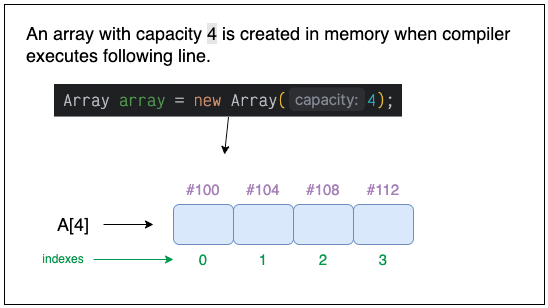
So our array is ready to be loaded with input data. Our array instance size is 4
, but we are inputting an array containing 9
elements.
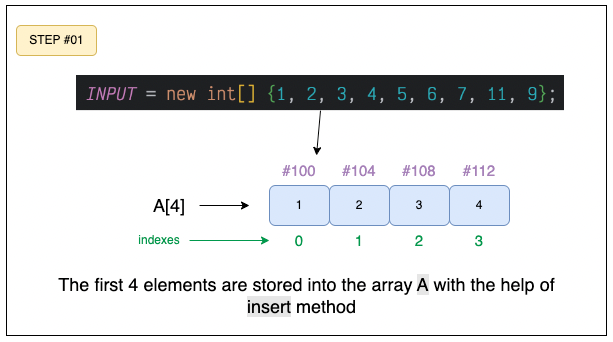
After adding the first 4
elements from the input array, our array has reached its maximum capacity. Now the insert
method doubles the array size and adds the next round of 4
elements. The following illustration explains.
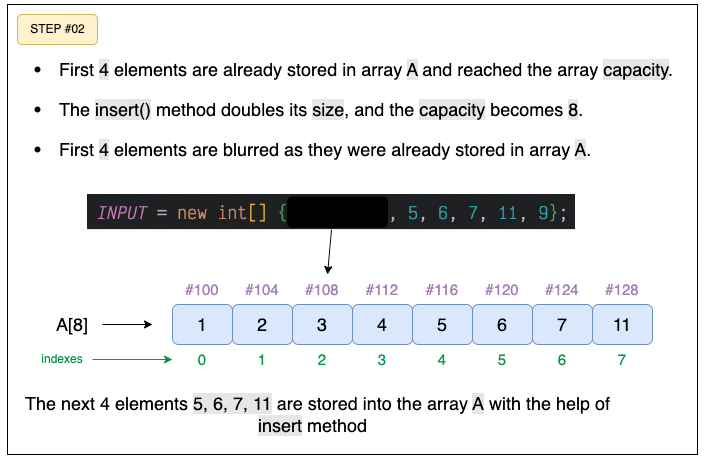
After adding the first 8
elements from the input array, our array has reached its maximum capacity again. Now the insert
method doubles the array size and adds the next round of 1
elements from the input array. The following illustration explains.
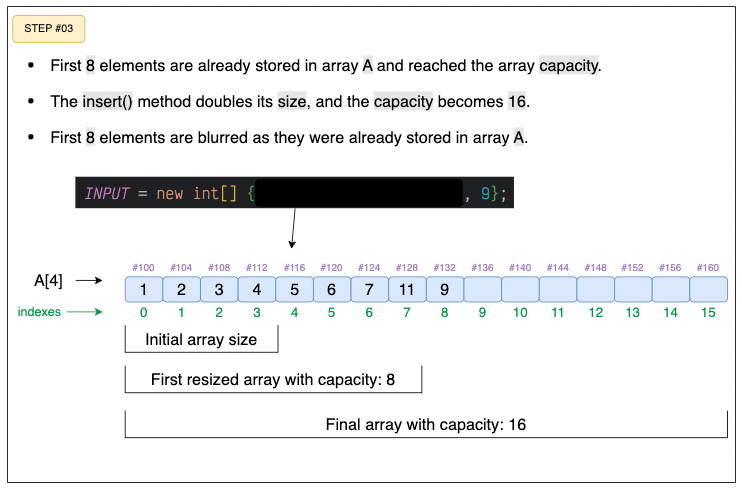
The insert
method doubles its size when the array is insufficient and there are extra elements to add. So the size increases from 4
to 8
, then from 8
to 16
.
Note: Do not get confused about thesize
variable and how it's doubling itself. Check theinsert
method that does this trick when the array is full. This is the reason you are seeing extra zeros forprint()
method in above code.
This concludes the lesson.